技术饭
微信支付、支付宝支付代码整理,仅供参考
微信支付、支付宝支付代码整理,仅供参考,作为个人技术开发一直很头疼微信支付、支付宝支付需要企业账号来做测试,所以很大程度上就没法完成测试,现在支付宝开放了沙箱功能算是很好了,但是微信支付还是一样不给沙箱测试,即使有沙箱测试也必须是企业账号。
1、支付
//支付代码调起支付
$pay = \pay\Pay::getInstance(
'wxpay', //支付类型
[
'notify_url' => url('course/payNotify', '', '', true) //配置参数
],
[
"body" => "课程订单",
'goods_name' => $order['goods_name'],
'pay_amount' => $order['pay_amount'],
'order_sn' => $order['order_sn'],
'order_id' => $order['id'],
'openid' => $userInfo['openid']
]
);
$res = $pay->pay();
2、支付代码
/**
* [pay 支付]
*/
public function pay($request_data = []) {
//合并数据
$request_data = $this->mergeRequestData($request_data);
//实例化模型
$input = new \WxPayUnifiedOrder();
//设置商品或支付单简要描述
$input->SetBody($request_data['body']);
//设置附加数据
$attach = json_encode(['order_id' => $request_data['order_id'], 'order_sn' => $request_data['order_sn']]);
$input->SetAttach($attach);
//设置订单流水号
$input->SetOut_trade_no($request_data['order_sn']);
//设置支付金额,订单总金额,单位为分
$input->SetTotal_fee($request_data['pay_amount']*100);
//设置设置订单生成时间
$input->SetTime_start(date("YmdHis"));
//设置商户号
$input->SetMch_id($this->options['mch_id']);
//设置appid
$input->SetAppid($this->options['appid']);
//设置订单失效时间
$input->SetTime_expire(date("YmdHis",time() + 600));
//设置商品标记,代金券或立减优惠功能的参数
$input->SetGoods_tag($request_data['body']);
//异步回调
$input->SetNotify_url($this->options['notify_url']);
//设置类型
$input->SetTrade_type("JSAPI");
//设置用户openid
$input->SetOpenid($request_data['openid']);
//配置类
$config = new \WxPayConfig($this->options);
//下单
$order = \WxPayApi::unifiedOrder($config, $input, 6);
if($order["return_code"] != "SUCCESS") {
$order['mch_id'] = $input->GetMch_id();
$order['appid'] = $input->GetAppid();
//返回数据
$rdata['code'] = 0;
$rdata['msg'] = $order['return_msg'];
$rdata['data'] = $order;
return $rdata;
} else if(!isset($order['prepay_id'])) {
//返回数据
$rdata['code'] = 0;
$rdata['msg'] = '预支付ID错误';
$rdata['data'] = $order;
return $rdata;
}
//预支付ID
$prepay_id = isset($order['prepay_id']) ? $order['prepay_id'] : '123456789';
$jsApiPay = new \WxPayJsApiPay();
$jsApiPay->SetAppid($this->options['appid']);
$jsApiPay->SetNonceStr($order['nonce_str']);
$jsApiPay->SetPackage('prepay_id=' . $prepay_id);
$jsApiPay->SetTimeStamp(time());
$jsApiPay->SetSignType('MD5');
$sign = $jsApiPay->SetSign($config);
$payInfo = [
'appid' => $this->options['appid'],
'noncestr' => $order['nonce_str'],
'sign' => $jsApiPay->GetSign(),
'package' => $jsApiPay->GetPackage(),
'timeStamp' => $jsApiPay->GetTimeStamp(),
'order_id' => $request_data['order_id'],
'order_no' => $request_data['order_sn'],
'notify_url' => $this->options['notify_url']
];
//返回数据
$rdata['code'] = 1;
$rdata['msg'] = '预支付成功';
$rdata['data'] = $payInfo;
return $rdata;
}
3、回调
/**
* [payNotify 异步通知]
*/
public function payNotify(){
//获取返回数据
$xml = file_get_contents("php://input");
//将文件转换成对象
$objectxml = simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
//将对象转换个JSON
$xmljson = json_encode($objectxml);
//将json转换成数组
$payinfo = json_decode($xmljson, true);
//file_put_contents('1.txt', json_encode($payinfo), FILE_APPEND);
//exit();
/*$params = $this->request->param();
//测试数据
$payinfo = [
'return_code' => 'SUCCESS',
'result_code' => 'SUCCESS',
'attach' => json_encode([
'order_id' => $params['order_id'],
'order_sn' => $params['order_sn']
]),
'out_trade_no' => $params['order_sn'],
'transaction_id' => '121775250120140703323233368018'
];*/
//验证状态码
if(empty($payinfo) || !isset($payinfo['return_code']) || !isset($payinfo['result_code'])){
exit();
}
//验证是否处理成功
if($payinfo['return_code'] == "SUCCESS" && $payinfo['result_code'] == "SUCCESS"){
//查询订单信息
$attach = json_decode($payinfo['attach'], true);
if(!isset($attach['order_id']) || !isset($attach['order_sn']) || $attach['order_sn'] != $payinfo['out_trade_no']){
exit();
}
//查询订单
$where = [];
$where[] = ['pay_status', '=' , 0];
$where[] = ['order_sn', '=' , $payinfo['out_trade_no']];
$where[] = ['id', '=' , $attach['order_id']];
$order = \Db::name('course_order')->where($where)->find();
if(empty($order)){
exit();
}
//查看用户信息
$userInfo = \Db::name('user')->field('id,nickname,score,openid')->where('status', '=', 1)->find($order['user_id']);
if(empty($userInfo)){
exit();
}
//开启事务
\Db::startTrans();
//1.订单支付成功
$course_order_update_data = [];
$course_order_update_data['id'] = $order['id'];
$course_order_update_data['pay_status'] = 1;
$course_order_update_data['pay_info'] = json_encode($payinfo);
$course_order_update_data['pay_time'] = date("Y-m-d H:i:s");
$course_order_update_data['pay_trade_no'] = $payinfo['transaction_id'];
$course_order_update_data['update_time'] = date("Y-m-d H:i:s");
$res_1 = \Db::name('course_order')->update($course_order_update_data);
//验证类型:支付类型(1.微信,2.积分,3.微信+积分)
$res_2 = 1;
$res_3 = 1;
if($order['pay_type'] == 3){
//积分扣除逻辑
//2.扣除用户积分
$update_data = [];
$update_data['id'] = $userInfo['id'];
$update_data['score'] = \Db::raw('score-'.$order['score']);
$update_data['update_time'] = date("Y-m-d H:i:s");
$res_2 = \Db::name('user')->update($update_data);
//3.记录用户积分变动
$add_data = [];
$add_data['user_id'] = $userInfo['id'];
$add_data['type'] = 2;
$add_data['score'] = $order['score'];
$add_data['score_balance'] = $userInfo['score'] - $order['score'];
$add_data['desc'] = "用户:".$userInfo['nickname'].",支付订单:".$order['goods_name'].",支付积分:".$order['score'];
$res_3 = \Db::name('user_score_log')->insert($add_data);
}
//验证结果
if($res_1 && $res_2 && $res_3){
// 提交事务
\Db::commit();
echo 'success';
exit;
} else {
// 回滚事务
\Db::rollback();
echo 'fail';
exit;
}
}
}
/**
* [refundNotify 退款异步通知]
*/
public function refundNotify(){
//获取返回数据
$xml = file_get_contents("php://input");
//将文件转换成对象
$objectxml = simplexml_load_string($xml, 'SimpleXMLElement', LIBXML_NOCDATA);
//将对象转换个JSON
$xmljson = json_encode($objectxml);
//将json转换成数组
$payinfo = json_decode($xmljson, true);
//微信支付
$pay = \pay\Pay::getInstance('wxpay', [], ["req_info" => $payinfo['req_info']]);
$payinfo['req_true_info'] = $pay->decodeRefundNotify();
//file_put_contents('1.txt', json_encode($payinfo), FILE_APPEND);
//file_put_contents('2.txt', json_encode($payinfo['req_true_info']), FILE_APPEND);
/* $params = $this->request->param();
//测试数据
$payinfo = [
'return_code' => 'SUCCESS',
'result_code' => 'SUCCESS',
'attach' => json_encode([
'order_id' => $params['order_id'],
'order_sn' => $params['order_sn']
]),
'out_trade_no' => $params['order_sn'],
'transaction_id' => '121775250120140703323233368018'
];*/
//验证状态码
if(empty($payinfo) || !isset($payinfo['return_code'])){
exit();
}
//验证是否处理成功
if($payinfo['return_code'] == "SUCCESS" && $payinfo['req_true_info']['refund_status'] == "SUCCESS"){
//查询订单
$where = [];
$where[] = ['pay_status', '=' , 1];
$where[] = ['order_sn', '=' , $payinfo['req_true_info']['out_trade_no']];
$order = \Db::name('course_order')->where($where)->find();
if(empty($order)){
exit();
}
//查看用户信息
$userInfo = \Db::name('user')->field('id,nickname,score,openid')->where('status', '=', 1)->find($order['user_id']);
if(empty($userInfo)){
exit();
}
//开启事务
\Db::startTrans();
//1.订单支付成功
$course_order_update_data = [];
$course_order_update_data['id'] = $order['id'];
$course_order_update_data['pay_status'] = 2;
$course_order_update_data['refund_id'] = $payinfo['req_true_info']['refund_id'];
$course_order_update_data['refund_info'] = json_encode($payinfo);
$course_order_update_data['refund_time'] = date("Y-m-d H:i:s");
$course_order_update_data['update_time'] = date("Y-m-d H:i:s");
$res_1 = \Db::name('course_order')->update($course_order_update_data);
//验证类型:支付类型(1.微信,2.积分,3.微信+积分)
$res_2 = 1;
$res_3 = 1;
if($order['pay_type'] == 3){
//积分返还逻辑
//2.返还用户积分
$update_data = [];
$update_data['id'] = $userInfo['id'];
$update_data['score'] = \Db::raw('score+'.$order['score']);
$update_data['update_time'] = date("Y-m-d H:i:s");
$res_2 = \Db::name('user')->update($update_data);
//3.记录用户积分变动
$add_data = [];
$add_data['user_id'] = $userInfo['id'];
$add_data['type'] = 1;
$add_data['score'] = $order['score'];
$add_data['score_balance'] = $userInfo['score'] + $order['score'];
$add_data['desc'] = "用户:".$userInfo['nickname'].",申请退款订单:".$order['goods_name'].",退款成功,返还积分:".$order['score'];
$res_3 = \Db::name('user_score_log')->insert($add_data);
}
//验证结果
if($res_1 && $res_2 && $res_3){
// 提交事务
\Db::commit();
echo 'success';
exit;
} else {
// 回滚事务
\Db::rollback();
echo 'fail';
exit;
}
}
}
4、代码参考
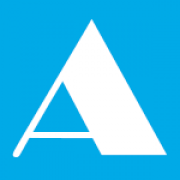
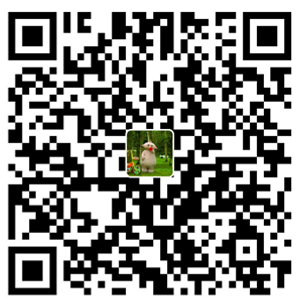
文明上网理性发言!